Wednesday, November 14, 2007
Methods and Properties
Methods are very useful in the C# language. Methods can help organize and simplify code, and are used all the time in game programming. This lesson should be able to introduce anyone who knows even nothing about programming, to the world of methods and how to create them, use them, and benefit from them in your programming.
A method can be looked at like a named container that holds code and does something to a value. This can be useful, for example, if you wanted to explode a ship. Instead of re-writing the code to explode the ship every time there is a collision, you can just create a method that explodes a ship, and then just call that method every time you want a ship to explode.
First, before we can call a method, we need to learn how to create methods. Here is the method creation structure:
accessModifier returnType methodName ( parameters )
First of all, methods need an access modifier. This modifier serves the same purpose as access modifiers on variables.
Next, there is the return type. The return type shows what type of variable you want the method to return. For example, let’s say that we have created a method called GiveBackEight. This method would look like the following:
private int GiveBackEight()
{
return 8;
}
When we call this method, we want to set any integer to a value of eight. In order to be able to set an integer to the value that the method returns we need to have an int as the parameter type. This way, we can set the value of a variable to the value this method returns by using the command int myVariable = GiveBackEight(); This sets the value of myVariable to the value of what GiveBackEight returns.
You can also create a variable that has a return type of, void which means the method does not return anything. These types of methods could be used for example to just draw something. You could declare a method that drew the number eight on the screen like this:
private void DrawEight()
{
Console.WriteLine("8");
}
This just draws the number eight onto the screen. These types of methods don’t give back anything. These types of methods are to do commands that will finish themselves. They are never used to change the value of a variable and give it back because with a void return type, the methods cannot return anything. You can call this method by using a command like DrawEight(); This just calls the method to do what it does.
After the return type, you have the method name, which is just like a variable name, and is used to access the method.
Finally, you have the parameters, which are placed in parentheses. A parameter is like a value that is given from an outside source so that the method can complete what it needs to do accurately. We have talked about these types of variables in the previous chapter. For example, if we wanted to allow the capability to draw any number, not just eight, we could change the DrawEight method to:
private void DrawNumber(int numberToDraw)
{
Console.WriteLine(numberToDraw.ToString());
}
This code takes any number that you choose as a parameter, and then draws that number. This is very helpful because, instead of having to make a billion different methods to draw every number, you can just create one method that has the capability to draw any number.
Method Overloads
Sometimes you may want to create the same method, but with maybe a few different parameters. An example of this is, maybe you have an explosion method that explodes at a given intensity value. You may also want to make it where the default intensity value is 100. You could do something like this:
private void ExlodeShip(int intensity)
{
//Explosion code
}
private void ExplodeShip()
{
ExplodeShip(100);
}
This code first creates the ExplodeShip method. This method takes an int parameter for the intensity of the explosion. Below that method though, we have another method with the same name. This method is an overload of the original ExplodeShip method but takes no parameters. Since 100 is the most common explosion intensity, for most cases the user will just pass in 100 for the intensity value. Instead of making him do that, we create an overload of ExplodeShip that takes no parameters but calls the one-parameter ExplodeShip version and passes in 100 because that is what the user wants. For more complicated methods, this can save a lot of time.
There are a few things you need to watch out for when using method overloads. You need to be careful not to have a continual loop. This could maybe happen when you on accident call the same method that you are in. For example calling ExplodeShip(); in the zero-parameter method would result in a continual loop because you are inside the method that you are calling.
Another thing is that you can’t have the method overload have the same parameter types. Even if one overload has int intensity and another method has int timeDelay, you can’t do this because the C# compiler (program that executes the code) will not be able to tell the difference. For it, both overloads have the same parameter types and it won’t know which method to call.
Properties
Properties are another type of code-block that you can use in C#. Some people may think properties are really confusing, but they aren’t at all, and are almost exactly the same as methods. You could look at a property like a method that doesn’t have parameters. To create a property, you would do something like this:
accessModifier returnType propertyName
You can see that this format is almost the same as the method format. We have an access modifier, a return type, and a property name. These all serve the same purpose as for methods.
There is one exception though. Besides the fact that properties can’t take parameters, they also can’t have the void return type. This is because properties are usually used a little differently than methods. Methods are more used to do things to values and then give the value back. Properties aren’t so much used that way. Properties are more used to give a value or set a value of something. This would explain why you can’t have the void return type because properties can’t just do something; they have to return a value.
To explain this, you could create this method which would return the value of ten:
private int Ten()
{
return 10;
}
You could also create the same thing using a property:
private int Ten
{
get
{
return 10;
}
}
The property would be the correct way to go. All this property does, is, when you try to access the value of what Ten has, the get part of the property is called. The only difference is that instead of calling myInt = Ten(); which is the method version, you call myInt = Ten; which doesn’t use the parentheses because there can be no parameters in a property.
Properties are mostly used for when you want to access a value in a class. For example the MathHelper class has a property called Pi which gives the value of PI.
Set Properties
Set properties are also types of properties that you can use. The way I will explain this is in a game example. Pretend you had a player class. Inside that player class you have a health property that allows access to the health. You would want someone to be able to access the health variable and change it to his liking. You could do this by using this code:
private int health;
public int Health
{
get
{
return health;
}
set
{
health = value;
}
}
First, we declare a private variable called health. This variable allows us to access health in our class when we want to change it or set it to a value.
After that we have our property called Health (remember variable names are case-sensitive). First we call the get part which returns the value of what our private health variable is. Then we call the set part. This part is called when we set the value of Health using something like Health = aValue;. In this part of the property we set health, our private variable, to value which is the value that we put on the right side of the equal sign when we set Health.
Since names in C# are case-sensitive, we can make a variable and a property have the same name. Of course, you may think that we can just make the private health variable into a
public one, we won’t have to worry about the property stuff. We could do this, but it would be incorrect programming practice. Also, using properties allows us to change the value of what we return which you can’t do if you just have a public variable. This could look like the following:
private int health;
public int TenMoreThanHealth
{
get
{
return health + 10;
}
set
{
health = value;
}
}
C# Primer
Variables--A quick primer on variables, how to create them, and how to modify their values.
Methods and Properties--Explains about how to create and call methods and properties.
I give all the desires of my heart to my Lord. May He work in everyone's heart so that they will discover the true joy that comes with following Him. Amen.
Variables
Creating Variables
Variables are very important in the C# language. Using variables gives you control of many different values. There are tons of different variables that can be used in C# and they all follow a similar pattern; one that we will explain.
First of all, what is a variable? A variable is named object that can be accessed and manipulated. You can access these values to use them in your project.
There are three different ways you can declare variables. The first way you can declare a variable is at the top of a class. This allows you to access the variable from any method or property inside of the class. These types of variables can be called class-variables. To declare a class variable, you would try and follow this format:
accessModifier variableType variableName ;
First of all, there is the access modifier. An access modifier is a keyword placed in front of a variable, method or other member, which restricts or allows access to it from some other class or struct. A member is any variable, property, or method inside of a class. The private modifier allows the least amount of access and allows the item to only be accessed inside the class it was created in. The protected modifier allows access only from inherited classes, and the public modifier allows total access from all classes.
There is also an access modifier named static. The static keyword allows the item it is being applied to, to not be part of a class instance but, instead, related to the class name. This modifier is not used at the moment but will be explained later.
After the access modifier we have the variable type. Each variable type contains different information needed for that variable type. For example, the int class contains information that holds integers. The string class contains information that holds text, and the bool class contains information that holds true or false values. Each variable type is different but most can be created the same way.
Then, after the variable type, you can name the variable. Variable names can be anything they want, but they are case-sensitive.
Finally, you have the semi-colon which you place to signify that you are done creating the variable.
As I told you, there are different ways that you can create a variable. We just looked at the class variable type. There is also another way to create variables. In this way, you create a variable inside a method or property. The difference between these types of variables is that they can only be accessed inside of that method or property. You create these types of variables the same way as a class variable, but you do not place an access modifier because you can’t access the variable outside of the function it was created in. This limit of access is called a variables scope. You would want to create this type of variable like so:
variableType variableName ;
Finally, there is one last way you can create variables. These types of variables are used inside method calls and are called parameters. For example if I called the, Multiply method of a Math class then I could pass in, 5 as an parameter. I wouldn’t name the variable because I can never access it again, and I don’t need any access modifiers, variable type name, or even a semi-colon. All I need is the value of the variable.
There are many different types of variables and their uses can be used in different ways. Here is a list giving a quick description of the variable types and their uses:
Variable Type; Containment Type; Constructor Type
Int32(int); (-)2,147,483,64(7-8); int intVar;
Int64(long); (-)9,223,372,036,854,775,80(7-8);long longVar;
Byte(byte); 255; byte byteVar;
UInt32(uint); 4,294,967,295; uint uintVar;
Uint64(ulong); 18,446,744,073,709,551,615; ulong ulongVar;
Single(float); (-)7 Digits; float floatVar
Double(double); (-)15-16 Digits; double doubleVar;
Decimal(decimal); (-)28-29 Digits; decimal decimalVar;
String(string); Text between quotes(“”); string stringVar;
Char(char); One Character between symbols(‘’);char charVar;
Boolean(bool); True or False; bool boolVar;
Random; Used for Randomizing; Random rnd = new Random()
The Constructor Type shows how you can create a variable of that type. The Random class inherits from the Object class instead of the Type class and needs to be created using the, new ClassType(...); format. This way calls the constructor of that variable. The constructor of a variable is the method that is called to create the variable.
Assigning Values to Variables
Assigning values to variables is also very important. In order to actually be able to use the variable in your project, you need a way to assign values to it.
The assignment operator is used for assigning values. An operator is a symbol that C# recognizes as a command to do something to a variable(s). You can assign variables using the following structure:
leftHandVar = rightHandValue ;
First, we place the name of the variable we want to assign a value to. If a variable does not yet have a specified value, then this process is called initializing. A variables initial value is the value it is first set to. You can also initialize a variable at the same time it is created like, private int intVar = 4; If you don’t initialize a variable it will either be set to a default value like zero, or will be set to null, which means no value.
Next we have the equal operator. This operator gets the preceding variable ready to be assigned a value.
Finally, we have the new value we want the variable to be set to.
You can also assign a variable to equal the value of another variable. This could look like, intVar = intVar2;
Modifying Variable Values
After you have assigned a value to a variable, you will probably want to be able to modify that value. For numbers, there are some of the normal math operators that can be used. These operators can be used as such:
int1 += 10;
int1 -= 10;
int1 *= 10;
int1 /= 10;
These operators change the values of variables. The += means the same thing as, int1 = int1 + 10; You can also modify the right hand value by doing something like, int1 += 10 * 3
How to Render a Sprite Using the SpriteBatch
To render a sprite (a 2D texture) there are three main steps that need to be followed. These steps are as follows:
- Add an image to your project so that it can be accessed in your code.
- Create a Texture2D variable in your project and load the texture image into that variable using the Content Pipeline.
- Draw the texture onto the screen with certain parameters that you would like to be applied to the texture.
First, before you can draw the texture, you need to be able to access the texture you want to draw. Through a system called the Content Pipeline, you can load different content files into your project and then access them using a ContentManager. Though this process is important, we first need to load an image into our project.
To load an image, you need to first navigate to your Solution Explorer (View / Solution Explorer). After this, right click on the name of your project and select Add / Existing Item.
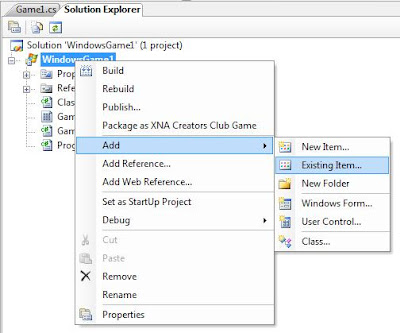
Figure 1: Right click on the name of your project and select Add / Existing Item
You should now be taken to a page where you can browse through your computer files. What you want to do is browse to where a simple texture is located. Once you have located where the file should be, in the Files of Type drop-down box, switch to Content Pipeline Files. This option shows all files that XNA will allow to be added to your project. Most likely, your image is of file type TGA, PNG, BMP, JPG, or DDS, all of which can be added into your project. Once your file is select, click Add. The file now should be added to your Solution Explorer where you can see it:
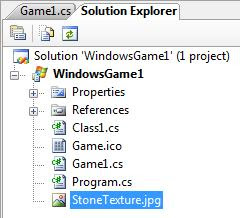
Figure 2: You can now see the texture file inside of your Solution Explorer. My texture was a JPG file called StoneTexture.
Creating a Variable to Hold the Texture
After loading an image into our project, we can now create a variable to contain that image data. This variable will then be used to draw the image onto the screen.
First, because our texture is a 2D image, we want to create a variable of type Texture2D. Create this variable at the top of the class near the GraphicsDeviceManager and ContentManager variables.
//The texture variable that will hold our image data.
Next, we need to initialize this variable. With most variables, you call the constructor of the class to create it. Unlike these classes, all content-related variables are created by directly loading them with a file. For our case, this will be the texture loaded into our project. Because of graphics device related issues which do not need to be explained in this lesson, put this code inside of the if (loadAllContent) statement of the LoadGraphicsContent method. This code initializes our Texture2D:
/*Use the ContentManager content variable to initialize
the Texture2D with a real texture.*/
texture = content.Load[Texture2D]
Code Note: The brackets in the above code should actually be tags. I needed to use brackets because the HTML would not allow tags.
This code statement may look long and confusing but it is quite simple. We call the Load method of a ContentManager variable named content (created by default). Then we specify for the method that we are loading a Texture2D and that the texture name is “StoneTexture” (for you that will probably be different). That is really all there is to that line.
Drawing the Texture Using the SpriteBatch
Finally, we have come to the part where we can display our texture. Using the SpriteBatch class and its Draw method, we can easily draw our texture.
Note: To draw a sprite means the same thing as to render a sprite.
The first step is to create the SpriteBatch. At the top of the class along with the other variable declarations, create a SpriteBatch:
//The SpriteBatch we will use to draw the texture.
SpriteBatch spriteBatch;
Now, we can initialize it. Inside of the Initialize method, add this code to initialize our SpriteBatch:
//Initialize the SpriteBatch.
spriteBatch = new SpriteBatch(graphics.GraphicsDevice);
The constructor for the SpriteBatch needs a GraphicsDevice. We access one by using the GraphicsDevice property inside of our GraphicsDeviceManager variable.
Since our SpriteBatch has now been created, we can now use it. The following calls to the SpriteBatch class will be placed in the Draw method right after the graphics.GraphicsDevice.Clear(Color.CornflowerBlue); line.
First, we need to start the SpriteBatch. The reason for doing this is to set any needed parameters for the SpriteBatch. These parameters are things such as allowing the ability to take transparency into account or setting the way the SpriteBatch will sort the sprites. Place this code into the Draw method:
/*Start the SpriteBatch. Your sprite may contain transparency so we allow Alpha-Blending.*/
spriteBatch.Begin(SpriteBlendMode.AlphaBlend);
Next, we want to draw the sprite. Place this code right after the previous line:
//Draw the sprite.
spriteBatch.Draw(texture, new Vector2(0, 0), Color.White);
We draw the sprite by calling the Draw method of the SpriteBatch variable. The first parameter is the Texture2D that we want to draw. We pass in texture. The next parameter is the position to draw the sprite at. This value is stored in a Vector2. For us, we specify 0, 0, which means that the origin of the sprite will be drawn at that position. The final parameter is the color to taint the background of the sprite with. We want the sprite to look completely normal so we specify Color.White.
Note: The default origin of the sprite is at the top-left corner of the sprite. To change this, a different overload of Draw needs to be called.
Finally, we need to “end” the SpriteBatch. There are two purposes for this. One is that instead of the sprites being drawn at the moment you call the Draw method, they are actually added to a list. Once End is called, all the sprites in the list are drawn at once. This is actually more efficient. The second reason is to reset the different values you changed when you called the Begin method. The different values are changed back to their default value until you call the Begin method again. After the two previous calls to the SpriteBatch, place this final statement:
//End the SpriteBatch.
spriteBatch.End();
Congratulations! You have now successfully coded a small application that renders a sprite onto the screen. Running the project now should result in your sprite drawn at the top-left corner of the screen (because we specified 0, 0 for the position).
Figure 3: After completing this lesson, you should hopefully see a result resembling this screen-shot.
I do hope that you have been able to solve some confusion by reading this lesson and I fully hope that you can easily continue along with your gaming quest. If you find any errors or mistakes, or if any parts of this lesson were confusing, please post a comment so that I can fix it. Thanks again.
Tuesday, November 13, 2007
Tutorials
The SpriteFont
How to Print Simple Text onto the Screen
2D Textures
How to Render a Sprite Using the SpriteBatch
I give all the desires of my heart to my Lord. May He work in everyone's heart so that they will discover the true joy that comes with following Him. Amen.
How to Print Simple Text onto the Screen
Most games need to be able to render text on the screen. Since many people would like to know how to do this, I’ve decided to write a small tutorial on this subject.
Text in itself is rendered onto the screen in the same way as a texture; through a bitmap image. On this image, every letter and symbol has its own small area. Before XNA 1.0 came out, I saw text being rendered through someone using their own system where a string is dissected and then each letter is drawn separately. Fortunately for us though, XNA 1.0 gives us the SpriteFont. This name refers both to an XML file and a C# class.
As said before, to render text follows almost the same concepts as rendering textures. The steps in the process are few and simple:
- Create a SpriteFont XML file and specify the needed properties.
- Load the SpriteFont file using the Content Pipeline, into a SpriteFont class.
- Render text using the specified SpriteFont and a shader (we use the SpriteBatch).
From these steps it is very easy to recognize the similarity between rendering textures and rendering text.
Creating the SpriteFont
So the first step to implementing a certain font is to create that font. This can be accomplished using the SpriteFont XML file. Inside of the Solution Explorer, right click on your project and click Add / New Item.
Figure 1: Right Click on your project name and click Add / New Item.
Note: A default Windows Game template is used with the namespace of WindowsGame1. It is not in the scope of this lesson to explain about project templates though.
You should now be taken to a page where you can choose what you want to create. We want to create a SpriteFont. Click on that template and name the SpriteFont, “Verdana”.
Figure 2: Create a SpriteFont named “Verdana”.
After clicking Add, in your Solution Explorer you will see the Verdana.spritefont file in your list. This file is already opened. If you take a quick look around the file you will notice several different XML tags specifying the different properties of the font. These properties are as follows:
- FontName: This property changes the font family of the font (i.e. Times New Roman, Verdana, etc.).
- Size: This property changes the size of the font.
- Spacing: This property changes the amount of spacing in between the characters of the text. Usually, you can leave this as the default value of two.
- Style: This property changes the appearance of your text. You can enter “Regular” for no changes; “Bold” for bolded text; “Italic” for italicized text; or finally, “Bold, Italic” for both bolded and italicized text. Unfortunately, there is not ability for underlined text.
For our simple purposes, we will leave the font properties as they are.
Note: If understanding the XML is confusing, do not worry. That is not necessary as long as you know what text you change. For all tags there is a common structure: [name]text[/endname]
Code Note: For the rest of the tutorial, open and close brackets will be used to replace open and close tags. I cannot use tags in the text due to the HTML problems.
Using the Content Pipeline to Create a SpriteFont Class
Now that we have our SpriteFont file created, we can load it into a SpriteFont class. This class will then be used to draw a specified string along with the help of the SpriteBatch class.
The first step is to create a variable for the SpriteFont. At the top of the class where the GraphicsDeviceManager and ContentManager are created, add this code:
//The SpriteFont to hold the Verdana XML font.
SpriteFont verdanaFont;
Next, we can initialize this variable. Similar to a Texture2D, instead of calling the constructor of the SpriteFont class (which is virtually useless) we instead directly load it with an outside file using content.Load(). We want to place this code into the LoadGraphicsContent method (so that it can be re-loaded when necessary), specifically inside of the if (loadAllContent) statement:
//Init the SpriteFont with a real SpriteFont file
//from the Solution Explorer.
verdanaFont = content.Load[Texture2D]
Code Note: Switch the two forward and backward slashes for tags. I couldn't use tags in this post because of the HTML. This code practice will be used for the rest of the tutorial.
This code first calls the Load method of the ContentManager variable content and tells it to load a SpriteFont. Then, we pass in the name of the SpriteFont XML file. Notice that we remove the file extension (i.e. .spriteFont).
Note: The reason why we load the SpriteFont inside of the LoadGraphicsContent method is not to be explained in this lesson. For now you don’t really need to know anyway.
Rendering Text onto the Screen Using the SpriteBatch
Finally, we have come to the part where we can render text onto the screen. As said earlier, a font is exactly the same in that it is a bitmap image that needs to be rendered. The SpriteBatch class, which is commonly used to render textures, can also be used along with its DrawString method to render text. Note that, the SpriteBatch actually draws the text. All the SpriteFont does is hold the value of what the text will look like.
Since we first need a SpriteBatch to begin drawing, add this code to the top of your class along with the other variable declarations. This code creates a SpriteBatch variable.
//The SpriteBatch used to draw the text.
Now we want to initialize this variable. Inside of the Initialize method, place this code:
//Initialize the SpriteBatch.
spriteBatch = new SpriteBatch(graphics.GraphicsDevice);
The SpriteBatch constructor needs a GraphicsDevice class in order to be initialized. The GraphicsDeviceManager graphics has a GraphicsDevice in it, so we just use that.
Finally, we can draw the text. Using the SpriteBatch class and the SpriteFont class, we can render any string that we want. Inside of the Draw method, after the graphics.GraphicsDevice.Clear(Color.CornflowerBlue); line, add this code.
/*Start the SpriteBatch. This call is required to set different options. For us, we don't need any special modes so we call the zero-parameter method.*/
spriteBatch.Begin();
/*Draw the font. We pass in the SpriteFont to use, the string to draw, the position, and the color of the text.*/
spriteBatch.DrawString(verdanaFont, "Look at me!!", new
Vector2(100, 100), Color.Black);
/*Finally, end the SpriteBatch. For better processing results, all things to draw are placed in a list. When we call End, we draw all these things at once. Also, we return the different changed parameters back to their default values.*/
spriteBatch.End();
This code may seem confusing, but it can be easily divided into three simple parts.
First, we start the SpriteBatch. This is needed so that we can set different render-states for the device such as alpha-blending (transparency), or the way you want sprites to be sorted.
Then, you draw the SpriteBatch. This is the most important part of the code. In this call, we pass in several different parameters. First, we pass in the SpriteFont to be used. For us we passed in our Verdana font. Next, there is the actual string to draw. This can be anything you want. After this we pass in the position. The position is stored as a Vector2. We pass in 100, 100, which is just a little off of the top-left corner of the screen. Note that the top, left corner of the first letter of the string will be drawn at 100, 100. To change this you need to change the origin of where the text is drawn. Finally, we have the color of the text which mostly explains its purpose by its name.
The last part of the code is the call to end the SpriteBatch. As mentioned in the comments, it would be very inefficient to render each sprite whenever a Draw call was made. For this reason, all sprites to be drawn are put into a list. When the End method is called, all the sprites in the list are drawn at once. Also, once all the sprites are drawn, the different render-states for the device are reset back to their original values.
The Final Results
Finally, we can see the results of our work. If you run the project (F5), you should see something very similar to this:
Figure 3: The results of our efforts. Simple text printed onto the screen.
Hopefully, now you will see, “Look at me!!” written onto the screen. If you do not see these results or if you are confused or found an error, please post a comment so that I can fix it. I do hope this helped you with in your programming quest.
Answer: To tint the color of a Texture2D when using the Draw call of a SpriteBatch, you need to change the Color parameter. For example, to tint the background of a sprite to the color orange, you would make this call in the Draw method:
spriteBatch.Draw(myTexture, new Vector2(0, 0), Color.Orange);
The Color.Orange parameter is the important parameter. This tells the SpriteBatch to draw the texture with an overall orange-ish background. An example of the same texture drawn twice is shown below:
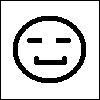
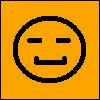
Figure 1: On the left there is the texture drawn with a white background. On the left, the same texture is drawn with an orange background.
The same texture was drawn once with Color.White. This value specifies that you want no changes to the background of the texture (i.e. if the original background was blue and you specified Color.White, the background would stay blue). Then the texture was drawn with Color.Orange. This drew the sprite with an orange-ish color. Note that if the original background was blue, the background would not turn orange, but instead the blue background would be mixed with the orange color to create a new, odd color.
Answer: Vibrating an Xbox 360 controller is actually quite simple. All you need to do is call the SetVibration method of the static GamePad class.
To vibrate a controller, you don’t actually need a GamePadState to work with because all you have to do is call GamePad.SetVibration(…). For our sake though, we will create a GamePadState just so that we can check if certain buttons are down and then vibrate the controller respectively. Create a GamePadState at the top of the Update method.
//The GamePad to check for input from.
GamePadState gamePad = GamePad.GetState(PlayerIndex.One);
Now, for a simple effect, whenever you are pressing the A button, the left motor of the controller vibrates and whenever you are pressing the B button, the right motor of the controller vibrates. To do this, place this code also into the Update method.
//Create two variables to keep track of the rotation values of the
//motors.
float leftMotorVibration = 0;
float rightMotorVibration = 0;
//If A is pressed, vibrate the left motor.
if (gamePad.Buttons.A == ButtonState.Pressed)
{
leftMotorVibration = 1;
}
else
{
leftMotorVibration = 0;
}
//If B is pressed, vibrate the right motor.
if (gamePad.Buttons.B == ButtonState.Pressed)
{
rightMotorVibration = 1;
}
else
{
rightMotorVibration = 0;
}
//Finally, vibrate the motors based on their individual values.
GamePad.SetVibration(PlayerIndex.One, leftMotorVibration,
rightMotorVibration);
First we create two simple floats to keep track of the vibration value of the motors. After that we check for input. Note that we have to check if the buttons are not pressed along with if they are pressed. This is because, if the buttons were pressed and then were released, nothing ever sets the vibration back to zero. Finally, after checking for input, we call the SetVibration method of the GamePad class. The first parameter specifies the player controller to vibrate which for us was Player 1. The second and third parameters specify the amount to rotate the motors by. For us we passed in leftMotorVibration and rightMotorVibration.
If you run the project now and press A or B, Player 1’s game controller should vibrate accordingly.
Question and Answer
The SpriteFont
What are all the possible font names that you can use for a SpriteFont?
The Xbox 360 Controller
How can I vibrate an Xbox 360 controller?
Drawing 2D Textures With the SpriteBatch
How do I tint the color of a Texture2D using the SpriteBatch?
I give all the desires of my heart to my Lord. May He work in everyone's heart so that they will discover the true joy that comes with following Him. Amen.
Question: What are all the possible font names that you can use for a SpriteFont?
Answer: Microsoft has allowed almost all the font families to be used for a SpriteFont. If you are having problems though, take not that there are some standards that need to be used in order to have XNA recognize the font family name.
- You need to allow spaces which may seem odd but is needed (i.e. the font family Times New Roman would still be entered in as “Times New Roman” and not “TimesNewRoman”).
- Also, obviously you need to spell the name correctly. No little mistakes as every letter matters. Also, there is no abbreviating.
- The Algerian font family is not accepted.